Published
- 3 min read
Why I prefer Arrow Functions over Regular Functions
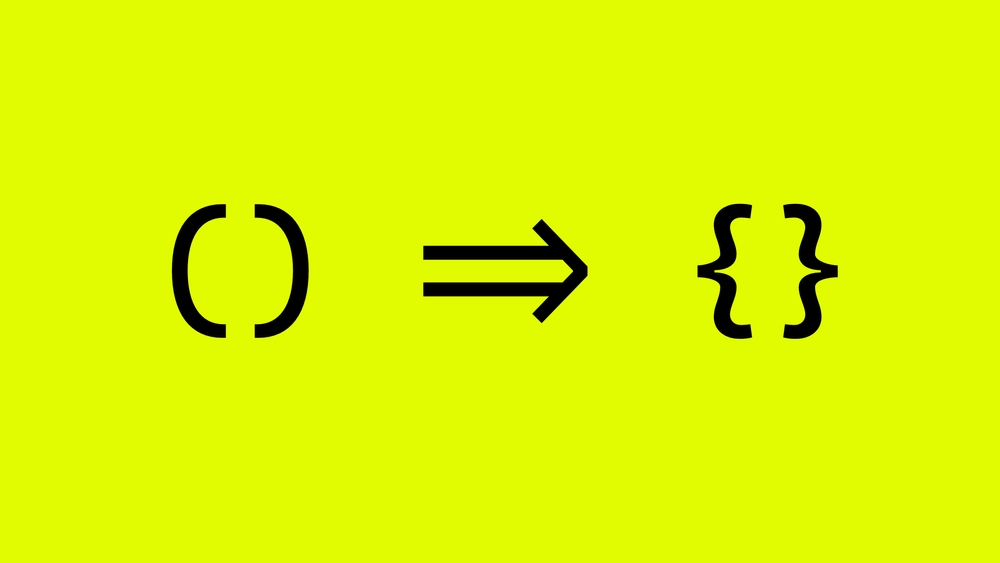
There are a couple of core differences between arrow functions and regular functions. Let’s explore why you should prefer writing all new code with arrow functions.
1. The ‘this’ Keyword
The this
keyword in JavaScript is a very confusing keyword. Depending on how you invoke the function the referenced object changes.
You can read all about it in the MDN Docs.
Of course, you can control it by using the bind-keyword and bind the context of the this
-keyword. In the end the problem remains that you have to be extra careful when using the keyword.
The real problem starts when you work with multiple people on a project. It is easy to introduce unintentional bugs when not every developer knows exactly how the keyword works.
Arrow functions, unlike regular functions - always references the outer context. It does not redefine the this keyword. This eliminates any confusion from the code, as you can be sure the keyword always references the same thing.
In production applications, you want to avoid using any global context. Thus, when you start using arrow functions, the usage of this
-keyword is reduced. Over time it disappears from the codebase which makes the code much easier to maintain.
2. The Arguments Object
Every function has a variable arguments
defined. This allows you to do the following:
function foo() {
console.log(arguments[0])
}
foo('Hello World') // This will print 'Hello World'
If you want to write easy to understand code you will want to avoid the arguments object. As the function signature does not suggest that you can pass any variable to it.
Arrow functions do not define the arguments object. It uses the variable from the outer context.
While the arguments
-variable is not very well known, and does not see a lot of usage. Not having to think about it makes it easier to reason about what a piece of code does.
3. The ‘new’ keyword
Regular functions can construct objects.
function Foo(value) {
this.value = value
}
const foo = new Foo('test')
I would put this in the category ‘interesting’ quirks of JavaScript. I have never seen such a code written in a practical application.
Modern JavaScript has the class keyword that makes it clear that you want to create an object.
Arrow functions cannot be used with the new
-keyword to create objects. Again, avoiding any confusion by design.
Conclusion
Arrow functions reduce the this
-keyword usage. There are no invisible features like the ‘arguments’ variable and it encourages you to use the class
keyword when you need to use objects.
I prefer using arrow functions because arrow functions create simpler and more readable code compared to regular functions.