Published
- 2 min read
CodeWars Kata: File Path Operations
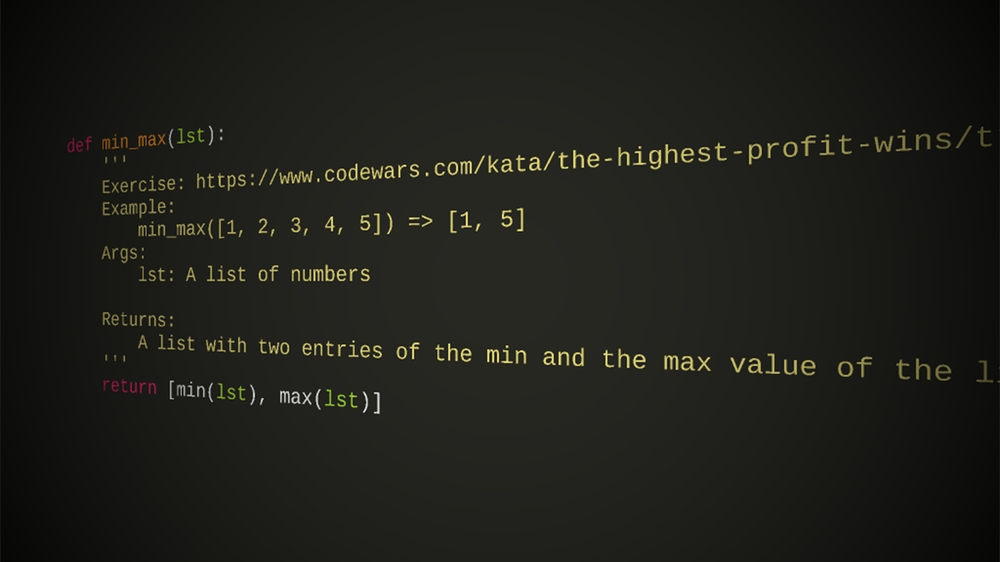
The problems in this Kata File-Path-Operations revolve around implementing common filename functions. Functions are needed to find the file-extension, the filename and the path to the directory.
To solve all three problems you need to find a specific pattern in a string. I choose to use Regular Expressions to solve the problems.
Regular Expressions are quite complicated to write. In order to quickly create and test regex expressions I use this website Regex 101. It allows you to quickly test your expression against multiple inputs as well as provides a documentation for the regex syntax.
Solution
export class FileMaster {
constructor(private filepath: string) { }
extension(): string {
return this.filepath.match(/\.(.*)/)[1];
}
filename(): string {
return this.filepath.match(/\/([^\/]*)\./)[1];
}
dirpath(): string {
return this.filepath.match(/(.*\/)/)[1];
}
}
Note
This code passed the test suite for the problem. However it is not suited for a production system.
The code does not work for these paths:
- Malformed filenames (invalid filepaths, cannot be detected)
- Files using path for Windows (Windows uses the ”- separator for paths)
- Does not work for folders containing a ’.’ (i.e.
.vscode/test.json
) - No handling of files without file extension
There are probably even more edge cases that I missed. Therefor in an production environment you should rely on the existing functions provided by the Path Module.